As I was reading 100 Go Mistakes and How to Avoid Them, I paused on the section about running linters. It illustrated the importance of linting by showing an example of variable shadowing in Go. I threw the code into VSCode and to my dismay… no linter warning!
5 minutes of Googling later I discovered that to lint for variable shadowing I’d need to install a few things:
- vet – a command line utility from Go’s standard library
- shadow – an analyzer that can detect shadowed variables
Install shadow from a terminal:
$ go install golang.org/x/tools/go/analysis/passes/shadow/cmd/shadow@latest
And to run vet with shadow enabled:
$ go vet -vettool=$(which shadow)
With vet and shadow up and running, time to boot up VSCode.
Install the Go Extension for VSCode
Get the Go extension for VSCode if you don’t have it already. The Go extension uses the staticcheck linter by default. We’re going to upgrade to a linter that can check for shadowed variables.
Enter golangci-lint
golangci-lint is a “Go linters aggregator”. It works by running a set of specified linters, like staticcheck, successively against your code. The default enabled linters are errcheck, gosimple, govet, ineffassign, staticcheck, and unused.
To install golangci-lint, you can follow the instructions on their website, or run the following in a terminal:
$ curl -sSfL https://raw.githubusercontent.com/golangci/golangci-lint/master/install.sh | sh -s -- -b $(go env GOPATH)/bin v1.55.2
Change the Go Lint Tool in VSCode Settings
In VSCode, open the settings and search for “go lint”:
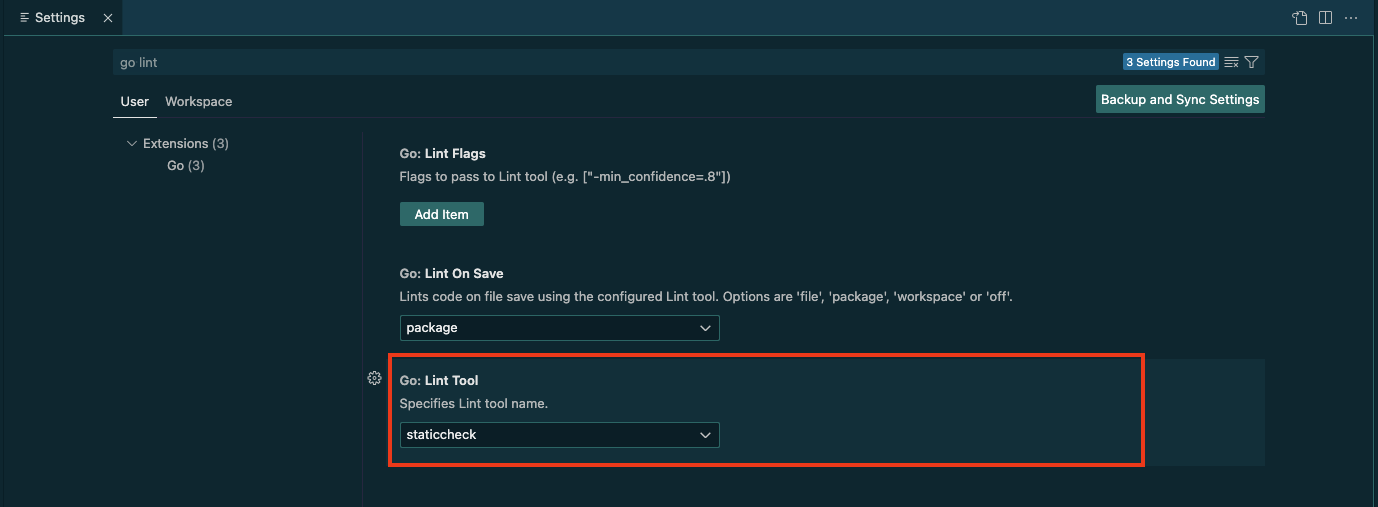
Change the highlighted input to “golangci-lint”:
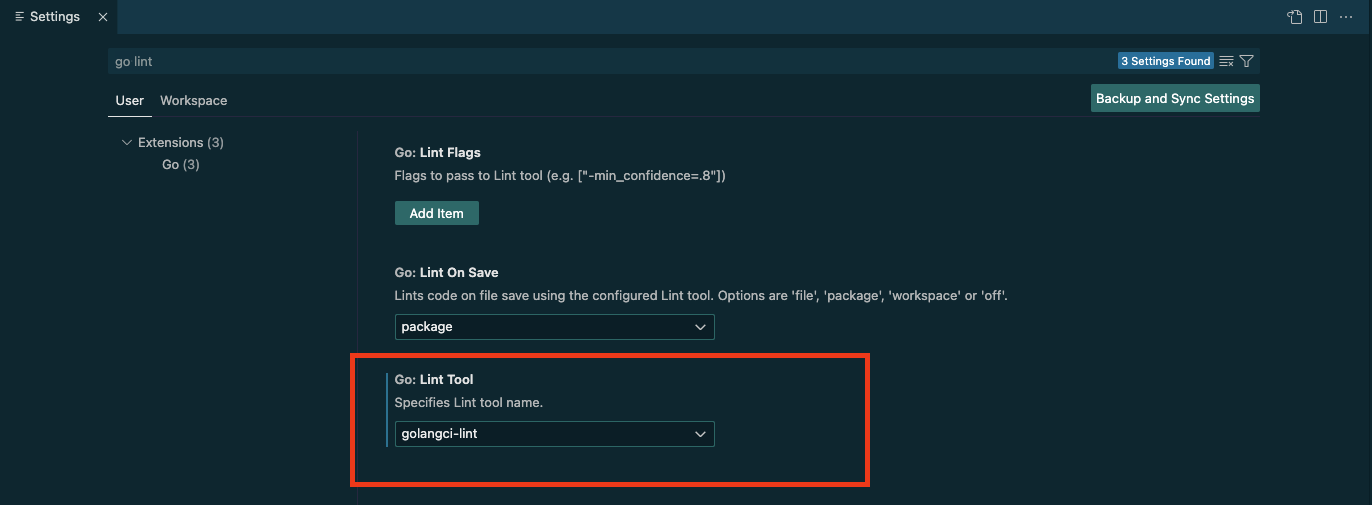
With the new lint tool in place, you may start seeing new lint warnings, but we’re not quite done yet. We still need to enable check-shadowing.
Enable check-shadowing
Our last step is to enable check-shadowing for govet to catch shadowed variables. To configure golangci-lint, first create a .golangci.yaml file either in the root of your project or in your home directory. To enable check-shadowing, add the following to your new config file:
linters-settings:
govet:
check-shadowing: true
This opens up the door for plenty of additional linter configurations offered by golangci-lint and all of its aggregate linters. You can read more about those here.
Fin
Reload the editor and run “Go: Lint Workspace” to potentially be bombarded with some happy lint accidents!